Paths
A path is s series of joined up lines that can be closed to form an irregular shape. It has its own constructor Path(), which creates a new path object, and it has a large number of methods. If path is the Path object these include,
path.moveTo( x, y) moves the path pointer to the position given by the float values x and y.
path.lineTo( x, y) draws a line to the point defined by the float values x and y.
path.close() completes the closed path by joining the last point drawn to the first one.
path.reset() clears the path and allows the variable to be reused to draw another path.
canvas.drawPath( path, paint) finally draw the path that has been defined by path using the current values of paint.
So here is an example of a path drawing operation to draw a diamond shape. The Path object has to be declared outside the onDraw() method. Otherwise a succession of draw movements would create new paths. So we just create on in the View class, which becomes. Also, the paint color has to be set separately.
public class ShapeView extends View{
Paint paint= new Paint();
Path path = new Path();
public ShapeView(Context context){
super(context);
}
public void onDraw(Canvas canvas){
float width=8;
float x=240,y=300, a=100, b=120;
this.setBackgroundColor(Color.LTGRAY);
path.moveTo(x-a, y); // first draw the path
path.lineTo(x, y-b);
path.lineTo(x+a, y);
path.lineTo(x, y+b);
path.close();
paint.setStyle(Paint.Style.STROKE); // draw the outline
paint.setStrokeWidth(width);
paint.setColor(Color.BLACK);
canvas.drawPath(path,paint);
paint.setStyle(Paint.Style.FILL); //fill the path
paint.setColor(Color.GREEN);
canvas.drawPath(path,paint);
}
}
The result of this code is as shown in Figure 1
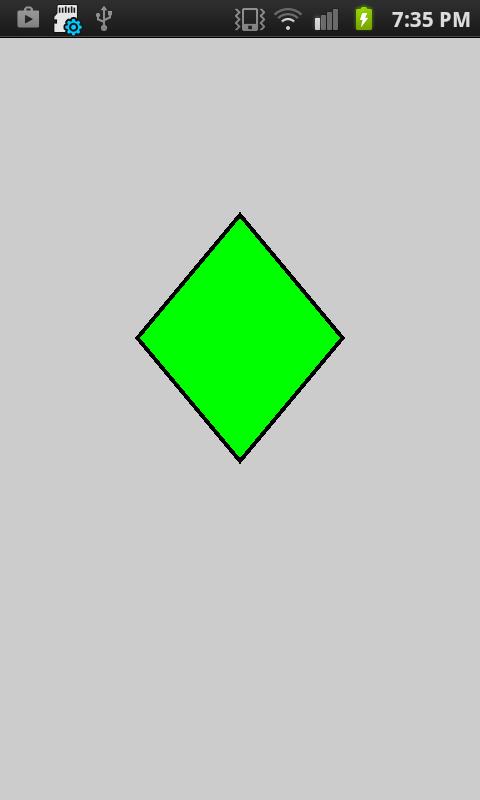